Example pie charts using python and matplotlib
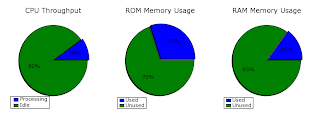
I needed to make some pie charts and didn't like the results I got from Excel. It was too hard to customize the plots exactly the way I wanted them. I have used Matlab before and I preferred Matlab to Excel. However, Python is my favorite thing to use so I searched for python and matlab on Google and found matplotlib. Matplotlib is a matlab-like plotting library for Python. You can get matplotlib from http://matplotlib.sourceforge.net/, but it is also bundled with the Enthought version of Python so I got it from there. Update: I realized that the Enthought bundle didn't include the latest version of matplotlib so I installed the latest version of matplotlib and the required NumPy as well.
Step-by-step:
1. Download enthought version of Python 2.4.3 from http://code.enthought.com/enthon/ (Click on the "enthon-python2.4-1.0.0.exe" link is at the bottom of the page) and install it.
2. Download "numpy-1.0.1.win32-py2.4.exe" from http://sourceforge.net/project/showfiles.php?group_id=1369 and install it.
3. Download "matplotlib-0.87.7.win32-py2.4.exe" from http://sourceforge.net/projects/matplotlib and install it.
3. Open a text editor and type this inside:
#!/usr/bin/env python
"""
http://matplotlib.sf.net/matplotlib.pylab.html#-pie for the docstring.
"""
from pylab import *
# create figure
figwidth = 10.0 # inches
figheight = 3.5 # inches
figure(1, figsize=(figwidth, figheight))
rcParams['font.size'] = 12.0
rcParams['axes.titlesize'] = 16.0
rcParams['xtick.labelsize'] = 12.0
rcParams['legend.fontsize'] = 12.0
explode=(0.05, 0.0)
colors=('b','g')
Ncols = 3
plotheight = figwidth/Ncols
H = plotheight/figheight
W = 1.0 / Ncols
margin = 0.1
left = [W*margin, W*(1+margin), W*(2+margin)]
bottom = H*margin
width = W*(1-2*margin)
height = H*(1-2*margin)
# cpu utilization
utilized = 10.0
free = 100.0 - utilized
fracs = [utilized, free]
axes([left[0], bottom, width, height])
patches = pie(fracs, colors=colors, explode=explode, autopct='%1.f%%', shadow=True)
title('CPU Throughput')
legend((patches[0], patches[2]), ('Processing', 'Idle'), loc=(0,-.05))
# ROM utilization
utilized = 30.0
free = 100.0 - utilized
fracs = [utilized, free]
axes([left[1], bottom, width, height])
patches = pie(fracs, colors=colors, explode=explode, autopct='%1.f%%', shadow=True)
title('ROM Memory Usage')
legend((patches[0], patches[2]), ('Used', 'Unused'), loc=(0,-.05))
# RAM utilization
utilized = 15.0
free = 100.0 - utilized
fracs = [utilized, free]
axes([left[2], bottom, width, height])
patches = pie(fracs, colors=colors, explode=explode, autopct='%1.f%%', shadow=True)
title('RAM Memory Usage')
legend((patches[0], patches[2]), ('Used', 'Unused'), loc=(0,-.05))
savefig('utilization')
show()
5. In Windows, go to Start -> All Programs -> Python 2.4 (Enthought Edition) -> IPython Shell
6. Type in "cd c:\temp"
7. Type "run piechart.py" and hit enter
Technorati tags: python, matplotlib, piechart
Related posts
- Creating a histogram plot with python — posted 2011-12-08
- How to draw a simple line using python and the matplotlib API - — posted 2007-01-05
- How to use the pylab API vs. the matplotlib API — posted 2007-01-04
- How to create some derived arrow classes with matplotlib and python — posted 2007-01-03
- How to draw an arrow with matplotlib and python — posted 2007-01-02
Comments
hi there,
Drawing of chart's has become very easy and amazing thanks to visifire
@Lita22,...
"Visifire is a set of data visualization controls - powered by Microsoft"
Are you kidding? :) on a blog on Emacs, Python and Ubuntu, he,he,he
Thanks for matplotlib demonstration.
You're close to have something that can draws any number of piechart.
left = [ (W*(id+margin) for id in xrange(NCols) ]